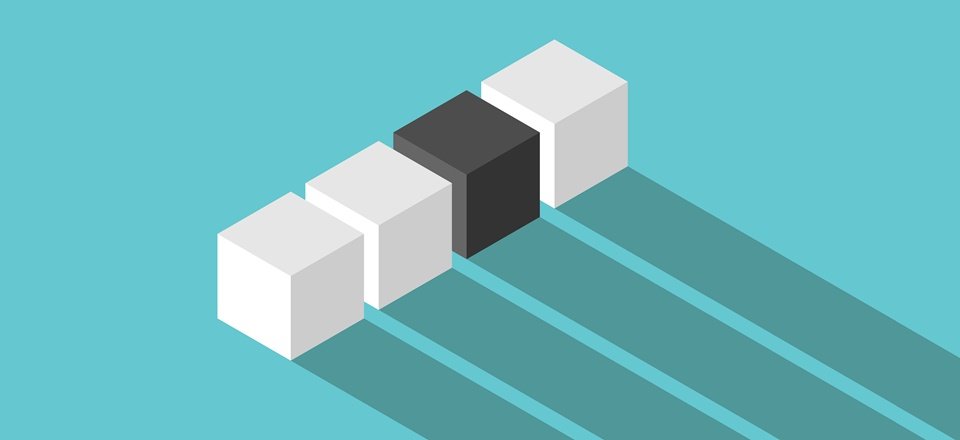
Introduction
This article will discuss how to compare adjacent elements in a List using Python. Suppose you want to create a new list from a given list by calculating the difference between adjacent elements of in the list, for example:
Input: test_list = [26, 19, 12, 6]
Output: new_list = [7, 7, 6]
Explanation:
26 – 19 = 7
19 – 12 = 7
12 – 6 = 6
There are several methods to achieve this. Each method demonstrated will use the same input list of [26, 19, 12, 6]
and produce the same output of [7, 7, 6]
Method 1: Using the range()
function:
def adjacent_items(seq):
new_list = []
for i in range(1, len(seq)):
previous_item = seq[i - 1]
current_item = seq[i]
new_list.append(previous_item - current_item)
return new_list # Output [7, 7, 6]
Method 2: Using the zip()
function and slices
:
def adjacent_items(seq):
new_list = []
list1 = seq[0::] # Copy of list starting at index 0
list2 = seq[1::] # copy of list starting at index 1
for previous_item, current_item in zip(list1, list2):
new_list.append(previous_item - current_item)
return new_list # Output [7, 7, 6]
Method 3: Using the zip()
function, slices
, and a list comprehension
:
new_list = [previous_item - current_item
for (previous_item, current_item)
in zip(test_list[0::], test_list[1::])]
print(new_list) # Output [7, 7, 6]
Method 4: Using a generator
and list comprehension
:
First lets create the generator.
def adjacent_items(seq):
iterable_list = iter(seq)
prev = next(iterable_list)
for item in iterable_list:
yield (prev - item)
prev = item
- Note: Since this is a
generator
, you must iterate over it to use it. We’ll use alist comprehension
to produce the results:
new_list = [item for item in adjacent_items(test_list)]
print(new_list) # Output [7, 7, 6]
Method 5: Using the more_itertool
and pairwise()
function:
- Note: You must use
pip
to installmore_itertools
pip install more_itertools
from more_itertools import pairwise
def adjacent_items(seq):
new_list = []
for previous_item, current_item in pairwise(lst):
new_list.append(previous_item - current_item)
return new_list # Output [7, 7, 6]
Of course the above example can be achieved using a list comprehension as well.
from more_itertools import pairwise
new_list = [previous_item - current_item
for (previous_item , current_item)
in pairwise(test_list)]
print(new_list) # Output [7, 7, 6]
For more information on more_itertools
visit Python docs.
Conclusion
The purpose of this article was to demonstrate how to compare adjacent elements in a List with Python. The code examples used in this article all create a new list from a given list by calculating the difference between adjacent elements of in the list. However, these functions can modify to suit your purpose. Hopefully you have a better understanding on how to compare adjacent elements in a List using Python. There are other solutions; these are just a few. If you found this article helpful or have any feedback, please leave a comment.