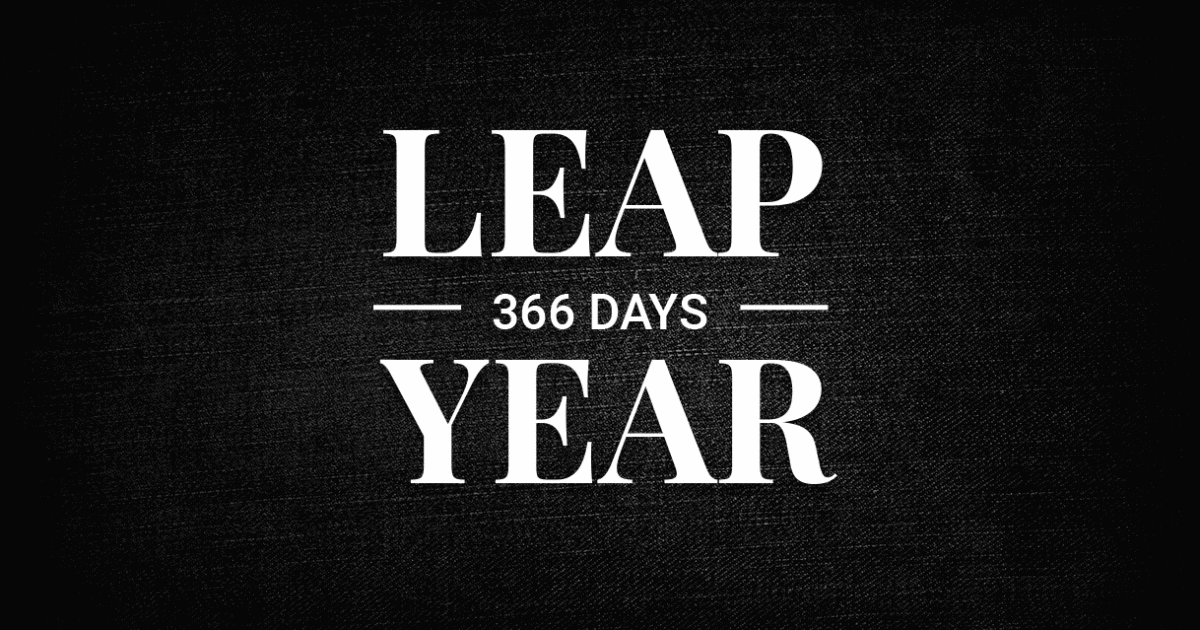
Introduction
The purpose of this article is to show how to calculate Leap Year using Python. Before delving into the code let’s discuss some background information on Leap Year what and why we have Leap Year.
What is a Leap?
A leap years a calendar year that contains an additional day added to keep the calendar year synchronized with the astronomical year or seasonal year. This is because it takes the Earth approximately 365.25 days to orbit the Sun — a solar year. The calendar year is usually rounded to 365. Therefore, to make up for the missing partial day, one day is added to our calendar approximately every four years (February has 29 days instead of 28). This at is a leap year.
The Algorithm
The following pseudocode is used to determine is a year is a Leap year or a common year.
if (year is not divisible by 4) then (it is a common year)
source: Wikipedia
else if (year is not divisible by 100) then (it is a leap year)
else if (year is not divisible by 400) then (it is a common year)
else (it is a leap year)
The approach I took to simplify this was:
If (year is divisible by 4) and (year is not divisible by 100) or (year is divisible by 400) then (it is a common year)
else (it is a common year).
def is_leap(year: str) -> bool:
"""Returns True if year is a Leap Year else False"""
CASE1: bool = year % 4 == 0
CASE2: bool = year % 100 == 0
CASE3: bool = year % 400 == 0
return CASE1 and not CASE2 or CASE3
if __name__ == '__main__':
is_leap(1955) # False
is_leap(1976) # True
This code can be simplified in a single line of code.
def is_leap(year: int) -> bool:
"""Returns True if year is a Leap Year else False"""
return year % 4 == 0 and not year % 100 == 0 or year % 400 == 0
if __name__ == '__main__':
is_leap(2013) # False
is_leap(2024) # True
Both code snippets will solve the HackerRank Leap Year Code Challenge. So this approach works.
Python Calendar Library
In Python, calendar.isleap()
is a function provided in calendar module that can be used to determine if a year is a Leap Year or a common year. This is probably the most simplest approach. Leap Year
import calendar
# checking whether given year is leap or not
print(calendar.isleap(2001)) # True
print(calendar.isleap(2016)) # False
Conclusion
Leap years are needed to keep our calendar in alignment with the Earth’s revolutions around the Sun. Hopefully after reading this post the Leap Year Algorithm has been demystified and you now know how to check if a year is a Leap Year or a common year using python.